Contact Form 7 Mailpoet Integration
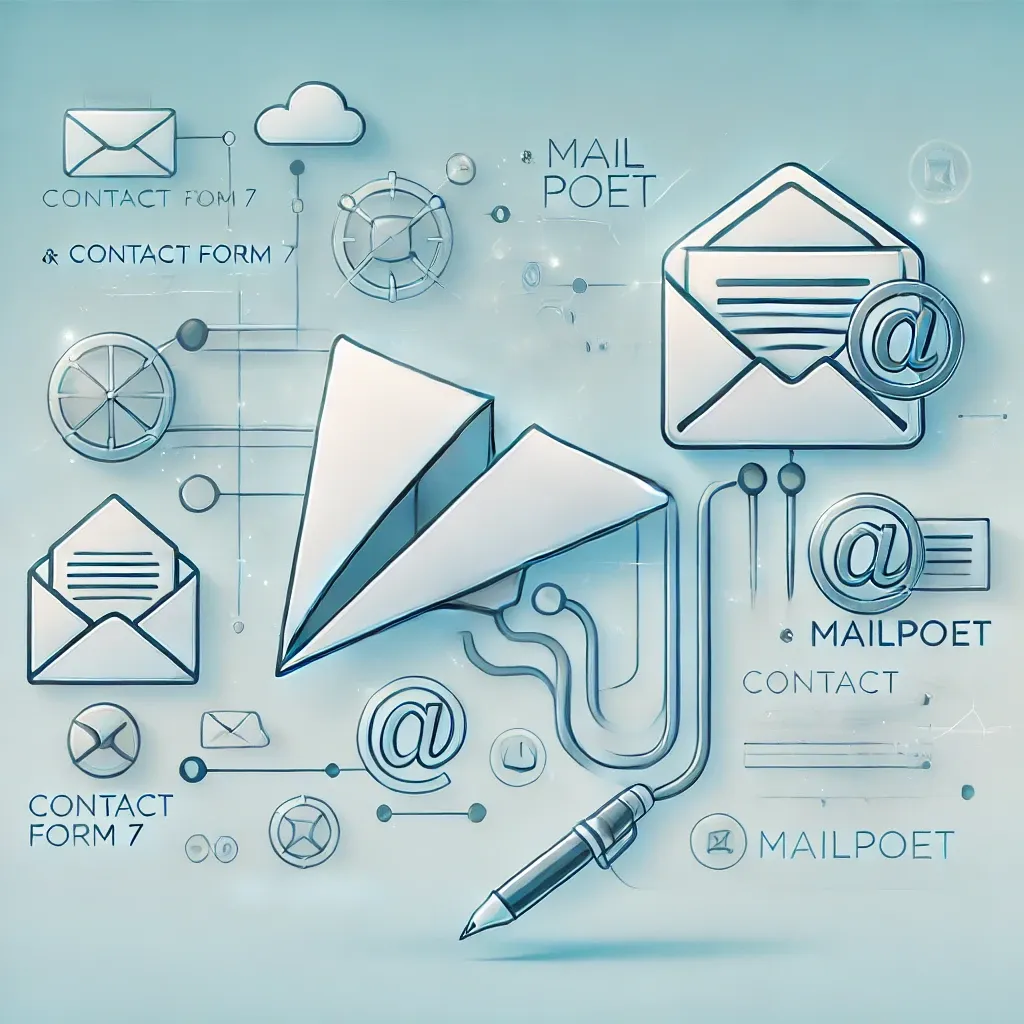
The plugin on the wordpress site stopped working, so I created one. Enjoy
<?php
/**
* Plugin Name: CF7 to MailPoet Integration
* Description: Adds Contact Form 7 submissions to a MailPoet subscriber list.
* Version: 1.0.9
* Author: DNA Levity
*/
// Log that the plugin is loaded only once
if (!defined('CF7_MAILPOET_PLUGIN_LOADED')) {
define('CF7_MAILPOET_PLUGIN_LOADED', true);
error_log('CF7 to MailPoet Integration plugin loaded.');
}
// Hook into Contact Form 7 submission
add_action('wpcf7_mail_sent', 'cf7_to_mailpoet_integration');
function cf7_to_mailpoet_integration($contact_form) {
// Log that the hook was triggered
error_log('CF7 to MailPoet Integration hook triggered (wpcf7_mail_sent).');
// Get the form ID dynamically and log it
$form_id = (string) $contact_form->id();
error_log('CF7 Form ID: ' . $form_id);
// Specify the form IDs you want to target (using numeric IDs as CF7 generally uses numbers)
$target_form_ids = [829, 955]; // Replace with your actual CF7 form IDs (numeric)
// Only proceed if the submitted form matches one of the targeted forms
if (!in_array($form_id, $target_form_ids)) {
error_log('Form ID ' . $form_id . ' does not match target forms.');
return;
}
// Get form submission data
$submission = WPCF7_Submission::get_instance();
if (!$submission) {
error_log('No submission data found.');
return;
}
$data = $submission->get_posted_data();
$email = isset($data['your-email']) ? sanitize_email($data['your-email']) : '';
$first_name = isset($data['your-name']) ? sanitize_text_field($data['your-name']) : '';
// Log email and name for debugging
error_log('Email: ' . $email . ', Name: ' . $first_name);
// Check if email is valid
if (!is_email($email)) {
error_log('Invalid email address: ' . $email);
return;
}
// Load MailPoet classes
if (!class_exists('\MailPoet\API\API')) {
error_log('MailPoet API class not found. Ensure MailPoet is installed and activated.');
return; // MailPoet is not installed or activated
}
$mailpoet = \MailPoet\API\API::MP('v1');
// Prepare subscriber data
$subscriber_data = [
'email' => $email,
'first_name' => $first_name,
];
// Set options for subscriber
$options = [
'send_confirmation_email' => true, // Disable confirmation email (set to true if needed)
'schedule_welcome_email' => false, // Disable welcome email (set to true if needed)
'skip_subscriber_notification' => true, // Disable admin notification email
];
try {
// Add subscriber to MailPoet list
$mailpoet->addSubscriber($subscriber_data, [3], $options); // Replace [3] with your MailPoet list ID(s)
error_log('Subscriber added successfully: ' . $email);
} catch (\MailPoet\API\MP\v1\APIException $e) {
// Handle specific MailPoet exceptions
switch ($e->getCode()) {
case 12:
error_log('Subscriber already exists: ' . $email);
break;
default:
error_log('MailPoet subscription error (code ' . $e->getCode() . '): ' . $e->getMessage());
}
} catch (Exception $e) {
// Handle any other exceptions
error_log('MailPoet subscription unexpected error: ' . $e->getMessage());
}
}
?>