Password Protected BIP84 Seed Phrase Cracker
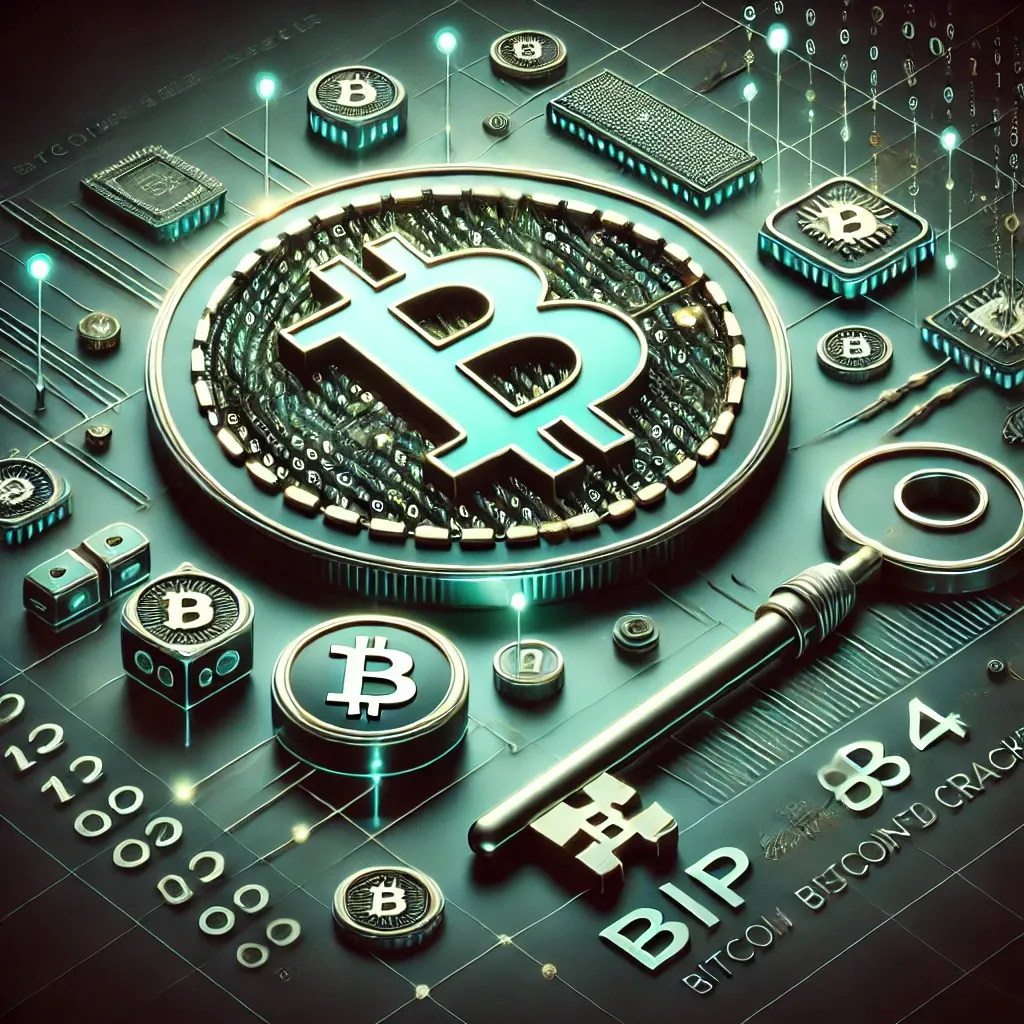
Samourai wallet no longer works because they got shut down by the US Government. Their block explorer api does not work, so their wallet shows an empty balance. A client ran into this issue and forgot her password.
I used chatgpt to make a password list and pasted it into this python script. It requires a free api key from Blockcypher to check the first address for transaction history. Enjoy!
import time
from blockcypher import get_address_overview
from bip_utils import Bip39SeedGenerator, Bip84, Bip84Coins, Bip44Changes
# Function to generate the first address and private key from a given BIP39 seed (BIP84 for bc1 address)
def generate_address_and_private_key_from_seed(seed):
bip84_mst = Bip84.FromSeed(seed, Bip84Coins.BITCOIN)
bip84_acc = bip84_mst.Purpose().Coin().Account(0).Change(Bip44Changes.CHAIN_EXT).AddressIndex(0)
return bip84_acc.PublicKey().ToAddress(), bip84_acc.PrivateKey().ToWif()
# Function to check if an address has transaction history using BlockCypher API
def has_transaction_history(address, api_key=None):
try:
# Using BlockCypher API to get address overview
overview = get_address_overview(address, coin_symbol='btc', api_key=api_key)
return overview.get('final_n_tx', 0) > 0 # Check if there are any transactions
except Exception as e:
print(f"Error checking transaction history for {address}: {e}")
return False
# Main function to check for transaction history using a list of possible passwords
def find_transaction_history_for_passwords(password_list, mnemonic, api_key=None):
for password in password_list:
try:
# Generate seed from mnemonic using password as a passphrase (not part of mnemonic)
seed_generator = Bip39SeedGenerator(mnemonic.strip())
seed = seed_generator.Generate(password.strip()) # Use password as passphrase here
# Generate address and private key from seed
address, private_key = generate_address_and_private_key_from_seed(seed)
print(f"Testing Address: {address} with Password: {password}")
# Check if the generated address starts with 'bc1' and if there is transaction history
if address.startswith("bc1") and has_transaction_history(address, api_key):
print(f"Address: {address} has transaction history.")
return {
"address": address,
"private_key": private_key,
"password": password
}
else:
print("No transaction history found for the given seed and password or address is not a bc1 address.")
# Introduce a delay of 1 second between requests to prevent hitting the rate limit
time.sleep(1)
except Exception as e:
print(f"Error generating address from seed with password '{password}': {e}")
return None
if __name__ == "__main__":
# Replace 'your_mnemonic_here' with the actual mnemonic to be tested
mnemonic = "your_mnemonic_here"
password_list = ["password", "password123", "Password123] # Replace with the list of possible passwords
api_key = "BlockCypher_API_key" # Replace with your BlockCypher API key if you have one
result = None
if api_key == "your_blockcypher_api_key":
print("Warning: No API key provided or using default placeholder. You may hit API rate limits.")
try:
result = find_transaction_history_for_passwords(password_list, mnemonic, api_key)
except Exception as e:
print(f"Unexpected error occurred: {e}")
if result is not None:
print(f"The address with transaction history is: {result['address']}")
print(f"The private key is: {result['private_key']}")
print(f"The password used is: {result['password']}")
else:
print("Could not find an address with transaction history for the provided mnemonic and passwords.")